Compare hkl_soleil E4CV with SPEC fourc#
Following the E4CV example, compare the orientation matix and positioning operations of hkl_soleil E4CV with SPEC fourc
Information from a SPEC data file will be used for the comparison.
In SPEC fourc geometry:
SPEC name |
mnemonic |
description |
---|---|---|
2theta |
tth |
Detector arm rotation |
Theta |
th |
Rotates sample circles |
Chi |
chi |
Sample tilt |
Phi |
phi |
Sample rotation |
The provided SPEC data file names these motors: tth
, th
, chi
, phi
so
this example will use the same names with the E4CV diffractometer to help the
comparison.
Read the SPEC scan from the data file#
The SPEC file provides all the information needed here. The spec2nexus (python) package can read the file and parse the content into useful structures, including deducing the diffractometer geometry in many cases.
import pyRestTable
from spec2nexus.spec import SpecDataFile
specfile = SpecDataFile("LNO_LAO_s14.dat")
specscan = specfile.getScan(14)
spec_d = specscan.diffractometer
spec_d.UB = spec_d.geometry_parameters["ub_matrix"][2]
terms = {
"SPEC file": specfile.specFile,
"scan #": specscan.scanNum,
"SPEC scanCmd": specscan.scanCmd,
"geometry": spec_d.geometry_name,
"mode": spec_d.mode,
"lattice": spec_d.lattice,
"wavelength": spec_d.wavelength,
"reflection 1": spec_d.reflections[0],
"reflection 2": spec_d.reflections[1],
"[UB]": spec_d.UB,
}
tbl = pyRestTable.Table()
tbl.labels = "term value".split()
for k, v in terms.items():
tbl.addRow((k, v))
print(tbl)
============ =================================================================================================================================================
term value
============ =================================================================================================================================================
SPEC file LNO_LAO
scan # 14
SPEC scanCmd hklscan 1.00133 1.00133 1.00133 1.00133 2.85 3.05 200 -400000
geometry fourc
mode Omega equals zero
lattice LatticeParameters3D(a=3.781726143, b=3.791444574, c=3.79890313, alpha=90.2546203, beta=90.01815424, gamma=89.89967858)
wavelength 1.239424258
reflection 1 Reflections3D(h=0.0, k=0.0, l=2.0, wavelength=1.239424258, angles=OrderedDict({'tth': 38.09875, 'th': 19.1335, 'chi': 90.0135, 'phi': 0.0}))
reflection 2 Reflections3D(h=1.0, k=1.0, l=3.0, wavelength=1.239424258, angles=OrderedDict({'tth': 65.644, 'th': 32.82125, 'chi': 115.23625, 'phi': 48.1315}))
[UB] [[-1.65871244e+00 9.82002413e-02 -3.89705578e-04]
[-9.55499031e-02 -1.65427863e+00 2.42844486e-03]
[ 2.62981891e-04 9.81574682e-03 1.65396181e+00]]
============ =================================================================================================================================================
Plot the (hkl) trajectories in the scan#
%matplotlib inline
import matplotlib.pyplot as plt
# plot the h, k, & l vs. point number
fig, axes = plt.subplots(3, 1, figsize=(12, 6))
fig.subplots_adjust(hspace=0.4, wspace=0.2)
plt.suptitle('Desired HKL trajectory')
axes[0].plot(specscan.data["H"])
axes[0].set_title("h")
axes[1].plot(specscan.data["K"])
axes[1].set_title("k")
axes[2].plot(specscan.data["L"])
axes[2].set_title("l")
plt.show()
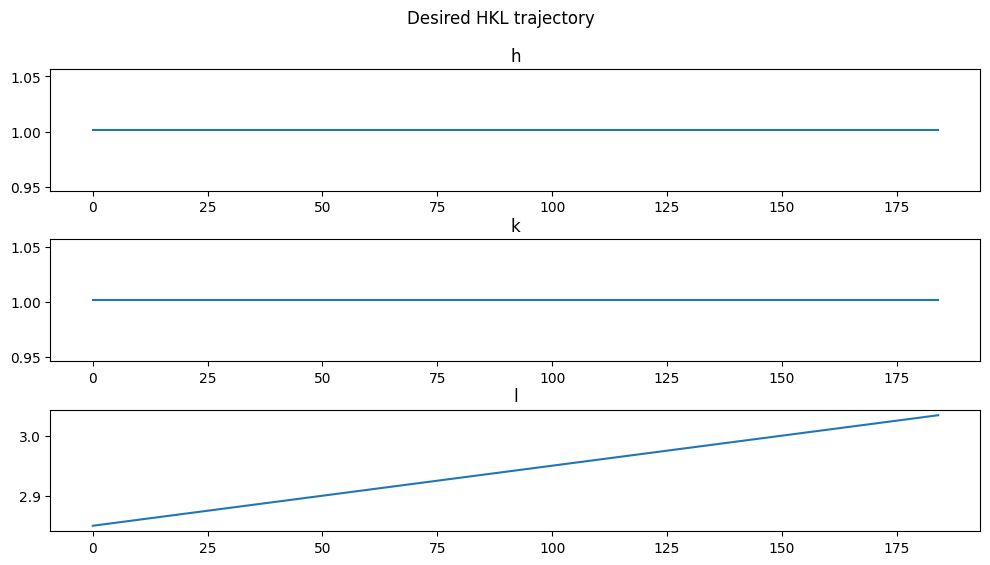
Setup the E4CV diffractometer in hklpy#
import hklpy2
fourc = hklpy2.creator(
name="fourc",
geometry="E4CV",
solver="hkl_soleil",
# use SPEC names for the axes and simulated motors (not EPICS)
reals=dict(th=None, chi=None, phi=None, tth=None),
)
# add the sample to the calculation engine
from hklpy2.user import add_sample, calc_UB, set_diffractometer, setor
set_diffractometer(fourc)
add_sample(
specfile.specFile, # sample name
a=spec_d.lattice.a,
b=spec_d.lattice.b,
c=spec_d.lattice.c,
alpha=spec_d.lattice.alpha,
beta=spec_d.lattice.beta,
gamma=spec_d.lattice.gamma,
)
Sample(name='LNO_LAO', lattice=Lattice(a=3.7817, b=3.7914, c=3.7989, alpha=90.2546, beta=90.0182, gamma=89.8997, system='triclinic'))
Test hklpy with the UB orientation matrix from SPEC#
Using the UB matrix as provided in the SPEC data file, compute the forward reflection positions and compare with those calculated by libhkl.
fourc.core.sample.UB
[[1.6614596270567616, 0.0, 0.0],
[0.0, 1.6614596270567616, 0.0],
[0.0, 0.0, 1.6614596270567616]]
# get the UB matrix from the SPEC data
# SPEC's UB first row moved (via numpy slicing) to last row for hklpy
fourc.core.sample.UB = spec_d.UB[[1,2,0], :]
print(f"{spec_d.UB=}")
print(f"{fourc.core.sample.UB}=")
# calculate angles with hklpy using the SPEC UB matrix
fourc.core.solver.mode = "bissector"
fourc.core.constraints["phi"].limits = (-50, 100)
fourc.core.constraints["tth"].limits = (-2, 180)
print(f"(002) : {fourc.forward(0, 0, 2)}")
print(f"(113) : {fourc.forward(1, 1, 3)}")
spec_d.UB=array([[-1.65871244e+00, 9.82002413e-02, -3.89705578e-04],
[-9.55499031e-02, -1.65427863e+00, 2.42844486e-03],
[ 2.62981891e-04, 9.81574682e-03, 1.65396181e+00]])
[[-9.55499031e-02 -1.65427863e+00 2.42844486e-03]
[ 2.62981891e-04 9.81574682e-03 1.65396181e+00]
[-1.65871244e+00 9.82002413e-02 -3.89705578e-04]]=
(002) : Hklpy2DiffractometerRealPos(th=15.262191782015, chi=89.914805456892, phi=99.115948808545, tth=30.52438356403)
(113) : Hklpy2DiffractometerRealPos(th=25.930677549264, chi=115.202910930796, phi=48.133061382025, tth=51.861355098529)
Define a custom reporting function to format the output table.
def add_ref_to_table(tbl, r):
sol = fourc.forward(r.h, r.k, r.l)
nm = f"{r.h:.0f} {r.k:.0f} {r.l:.0f}"
# print(nm, sol)
for sm in fourc.real_axis_names:
row = [f"({nm})", sm]
v_hklpy = getattr(sol, sm)
v_spec = r.angles[sm]
row.append(f"{v_hklpy:.5f}")
row.append(f"{v_spec:.5f}")
row.append(f"{v_hklpy-v_spec:.5f}")
tbl.addRow(row)
For each of the orientation reflections used in the SPEC file, report the computed motor positions for each reflection for E4CV and SPEC. We’ll only pick positions where $2\theta\ge 0$.
# Compare these angles with those from SPEC
tbl = pyRestTable.Table()
tbl.labels = "(hkl) motor E4CV SPEC difference".split()
r1, r2 = spec_d.reflections
fourc.core.solver.mode = "bissector"
fourc.core.constraints["tth"].limits = (-2, 180)
add_ref_to_table(tbl, r1)
# print(r2)
add_ref_to_table(tbl, r2)
print(tbl)
======= ===== ========= ========= ==========
(hkl) motor E4CV SPEC difference
======= ===== ========= ========= ==========
(0 0 2) th 15.26219 19.13350 -3.87131
(0 0 2) chi 89.91481 90.01350 -0.09869
(0 0 2) phi 99.11595 0.00000 99.11595
(0 0 2) tth 30.52438 38.09875 -7.57437
(1 1 3) th 25.93068 32.82125 -6.89057
(1 1 3) chi 115.20291 115.23625 -0.03334
(1 1 3) phi 48.13306 48.13150 0.00156
(1 1 3) tth 51.86136 65.64400 -13.78264
======= ===== ========= ========= ==========
Note that the angles do not match between E4CV and SPEC, even if we re-arrange the rows as we did above. Can’t just use the UB matrix from the one program in the other software.
Need to add the orientation reflections (with wavelength), then compute the UB matrix. Follow in the section below.
Setup the UB orientation matrix using hklpy#
Compute the UB matrix using hklpy (& libhkl).
fourc.wavelength.put(1.239424258) # Angstrom
refs = [
setor(
r.h, r.k, r.l,
tth=r.angles["tth"],
th=r.angles["th"],
chi=r.angles["chi"],
phi=r.angles["phi"],
)
for r in spec_d.reflections
]
UB = calc_UB(*refs)
tbl = pyRestTable.Table()
tbl.labels = "term value".split()
tbl.addRow(("SPEC [UB]", spec_d.UB))
tbl.addRow(("E4CV [UB]", UB))
print(tbl)
========= ==========================================================================================================================================================
term value
========= ==========================================================================================================================================================
SPEC [UB] [[-1.65871244e+00 9.82002413e-02 -3.89705578e-04]
[-9.55499031e-02 -1.65427863e+00 2.42844486e-03]
[ 2.62981891e-04 9.81574682e-03 1.65396181e+00]]
E4CV [UB] [[-0.095549863427, -1.654278751664, 0.002428444976], [0.000263111155, 0.009815859008, 1.653961890838], [-1.658712537638, 0.098200262676, -0.000389705597]]
========= ==========================================================================================================================================================
Report the results, as before, and compare with table above.
fourc.sample.lattice.crystal_system
'triclinic'
tbl = pyRestTable.Table()
tbl.labels = "term value".split()
tbl.addRow(("energy, keV", fourc._source.energy))
tbl.addRow(("wavelength, angstrom", fourc._source.wavelength))
tbl.addRow(("position", fourc.position))
tbl.addRow(("sample name", fourc.sample.name))
tbl.addRow(("lattice", fourc.sample.lattice))
tbl.addRow(("crystal system", fourc.sample.lattice.crystal_system))
tbl.addRow(("[U]", fourc.sample.U))
tbl.addRow(("[UB]", fourc.sample.UB))
print(tbl)
==================== ==========================================================================================================================================================
term value
==================== ==========================================================================================================================================================
energy, keV 10.003370326044433
wavelength, angstrom 1.239424258
position Hklpy2DiffractometerPseudoPos(h=0, k=0, l=0)
sample name LNO_LAO
lattice Lattice(a=3.7817, b=3.7914, c=3.7989, alpha=90.2546, beta=90.0182, gamma=89.8997, system='triclinic')
crystal system triclinic
[U] [[-0.057509496764, -0.998327391025, 0.005922677679], [0.000158361191, 0.005923373917, 0.999982444127], [-0.998344946751, 0.057509425056, -0.000182553939]]
[UB] [[-0.095549863427, -1.654278751664, 0.002428444976], [0.000263111155, 0.009815859008, 1.653961890838], [-1.658712537638, 0.098200262676, -0.000389705597]]
==================== ==========================================================================================================================================================
# Compare these angles with those from SPEC
# fourc.calc["phi"].limits = (-1, 100)
tbl = pyRestTable.Table()
tbl.labels = "(hkl) motor E4CV SPEC difference".split()
r1, r2 = spec_d.reflections
fourc.core.constraints["tth"].limits = (-2, 180)
fourc.core.solver.mode = "constant_phi"
fourc.phi.move(0)
add_ref_to_table(tbl, r1)
fourc.core.solver.mode = "bissector"
add_ref_to_table(tbl, r2)
print(tbl)
======= ===== ========= ========= ==========
(hkl) motor E4CV SPEC difference
======= ===== ========= ========= ==========
(0 0 2) th 19.12616 19.13350 -0.00734
(0 0 2) chi 90.01350 90.01350 -0.00000
(0 0 2) phi 0.00000 0.00000 0.00000
(0 0 2) tth 38.08407 38.09875 -0.01468
(1 1 3) th 32.81850 32.82125 -0.00275
(1 1 3) chi 115.20291 115.23625 -0.03334
(1 1 3) phi 48.13305 48.13150 0.00155
(1 1 3) tth 65.63700 65.64400 -0.00700
======= ===== ========= ========= ==========
SPEC data file#
%pycat LNO_LAO_s14.dat
#F LNO_LAO
#E 1276730676
#D Wed Jun 16 18:24:36 2010
#C LNO_LAO User = epix33bm
#H0 SR_current SR_fill SR_status SR_mode SR_fb SR_fbH SR_fbV SR_topUp barometer_mbar
#H1 SR_BM_HPOS SR_BM_VPOS SR_BM_HANG SR_BM_HANG
#H2 slits_wt slits_wl slits_wb slits_wr
#H3 Mir1_alpha Mir1_y Mir1_y1 Mir1_y2 Mir1_bender
#H4 DCM_energy DCM_lambda DCM_theta0 DCM_thetaEnc DCM_mode Mir_use Mir_alpha
#H5 Mir2_alpha Mir2_y Mir2_y1 Mir2_y2 Mir2_bender
#H6 mue_sclr_auto mue_sclr_freq
#H7 PF4_thickAl PF4_thickTi PF4_trans PF4_bladeA1 PF4_bladeA2 PF4_bladeA3 PF4_bladeA4 PF4_bladeB1 PF4_bladeB2 PF4_bladeB3 PF4_bladeB4
#H8 I0_VDC I0_gain I0_bias I0_time I0_suppr I0_dark I0_Amps
#H9 I00_VDC I00_gain I00_bias I00_time I00_suppr I00_dark I00_Amps
#H10 MUE540_i0 MUE540_i1 MUE540_i2 MUE540_i3 MUE540_i4 MUE540_i5 MUE540_i6 MUE540_i7
#H11 MUE540_i8 MUE540_i9 MUE540_i10 MUE540_i11 MUE540_i12 MUE540_i13 MUE540_i14 MUE540_i15
#H12 MUE540_o0 MUE540_o1 MUE540_o2 MUE540_o3
#H13 HSC_I0h HSC_I0v
#H14 HSC1t HSC1l HSC1b HSC1r HSC1h HSC1v HSC1h0 HSC1v0
#H15 HSC2t HSC2l HSC2b HSC2r HSC2h HSC2v HSC2h0 HSC2v0
#H16 HSC3t HSC3l HSC3b HSC3r HSC3h HSC3v HSC3h0 HSC3v0
#H17 dark1 dark2 dark3 dark4 dark5 dark6 dark7 dark8
#H18 dark9 dark10 dark11 dark12 dark13 dark14 dark15 dark16
#H19 darkToTime darkCoTime darkRing
#H20 powderMode powderWidth powderMotor powderVelo
#H21 rockON rockCenter rockWidth
#O0 2-theta theta chi phi antheta an2theta z-axis m_1_8
#O1 xt1 yt1 yt2 yt3 m_2_5 zt1 samx samz
#O2 DCM.theta wst wsl wsb wsr m22
#C Wed Jun 16 18:25:04 2010. do ./matrix150.mac.
#C Wed Jun 16 18:26:02 2010. Mode reset from 5 to 0.
#C Wed Jun 16 18:26:18 2010. Mode reset from 0 to 3.
#C Wed Jun 16 18:27:18 2010. Freezing Phi at 0.
#C Wed Jun 16 18:27:39 2010. do ./align.mac.
#S 14 hklscan 1.00133 1.00133 1.00133 1.00133 2.85 3.05 200 -400000
#D Wed Jun 16 18:47:18 2010
#M 400000 (I0)
#G0 0 0 1 0 0 1 0 0 0 0 0 0 50 0 0.1 0 68 68 50 -1 1 1 3.13542 3.13542 0 463.6 838.8
#G1 3.781726143 3.791444574 3.79890313 90.2546203 90.01815424 89.89967858 1.661462253 1.657219786 1.65396364 89.74541108 89.98229138 90.10024173 0 0 2 1 1 3 38.09875 19.1335 90.0135 0 0 0 65.644 32.82125 115.23625 48.1315 0 0 1.239424258 1.239424258
#G3 -1.658712442 0.09820024135 -0.000389705578 -0.09554990312 -1.654278629 0.00242844486 0.0002629818914 0.009815746824 1.653961812
#G4 1.001328179 1.001328179 2.999452893 1.239424258 29.28146688 29.4104177 0 89.84344949 0.7 0 0 0 0 0 0 0 -180 -180 -180 -180 -180 -180 -180 -180 -180 0
#Q 1.00133 1.00133 2.99945
#P0 65.644 32.82125 115.23625 48.1315 -0.001 -0.16 2.5 -0.2
#P1 -3.4480499 0.49927508 0.030010083 0.499275 1.749425 -113.52071 -946.48814 0
#P2 11.508019 2.9999781 30.000019 2.9999781 29.999854 1230.0415
#C LNO_LAO
#V0 102.36 4 1 4 1 1 1 1 986.893
#V1 -0.695912 0.254987 11.1785 11.1785
#V2 2.99998 30 2.99998 29.9999
#V3 5.50001 -6.18921e-06 3.7125 -3.71251 16
#V4 9.99906 1.23942 0.149966 11.4038 0 1 5.50001
#V5 5.78578 29.8071 33.7125 25.9017 15.2093
#V6 0 16960
#V7 0 0 1 0 0 0 0 0 0 0 0
#V8 4.98413 100000000 0 1 5.23e-07 785.198 4.98413e-08
#V9 2.78144 1000000 0 1 -2.9e-09 1 2.78144e-06
#V10 0.129426 2.78144 4.98413 0.01221 -0.0610501 -0.0610501 0.119658 -0.031746
#V11 -0.0757021 -0.0512821 0.393162 0.031746 -0.021978 -0.031746 -0.0512821 0.026862
#V12 5 5 5 0
#V13 2.1 0.5
#V14 0.9 1.7 1.1 2.3 4 2 0.3 -0.1
#V15 9.5 9.5 9.5 9.5 19 19 0 0
#V16 0.5 1.1 0.5 1.4 2.5 1 0.15 0
#V17 1000000 785.198 1 0.09375 0.0520833 1.39583 7.20833 203185
#V18 0 0 0 0 0 0 0 0
#V19 216 97 100
#V20 0 0 theta 0.4
#V21 OFF 0 0
#N 27
#L H K L Epoch I0 I00 harmonic W Fluor corrdet filters trans piltot TempC ccdtot scan_bar Energy SampK ContK vortot livet realt icr ocr sca1 seconds signal
1.00133 1.00133 2.85 1387 400000 217383 0 2030 2070 1989 0 1 0 0 0 157633 9.9990631 0 0 0 0 0 0 0 0 0.780109 1989
1.00133 1.00133 2.851 1390 400000 220156 0 2150 2186 2112 0 1 0 0 0 163578 9.9990631 0 0 0 0 0 0 0 0 0.79094 2112
1.00133 1.00133 2.852 1393 400000 219835 1 2210 2245 2163 0 1 0 0 0 161868 9.9990631 0 0 0 0 0 0 0 0 0.789425 2163
1.00133 1.00133 2.853 1396 400000 216589 1 2313 2353 2261 0 1 0 0 0 157376 9.9990631 0 0 0 0 0 0 0 0 0.777549 2261
1.00133 1.00133 2.854 1399 400000 215071 0 2225 2285 2176 0 1 0 0 0 156314 9.9990631 0 0 0 0 0 0 0 0 0.772036 2176
1.00133 1.00133 2.855 1402 400000 214735 2 2389 2440 2331 0 1 0 0 0 154578 9.9990631 0 0 0 0 0 0 0 0 0.771171 2331
1.00133 1.00133 2.856 1406 400000 212312 2 2370 2413 2317 0 1 0 0 0 152642 9.9990631 0 0 0 0 0 0 0 0 0.762595 2317
1.00133 1.00133 2.857 1409 400000 212443 1 2621 2673 2564 0 1 0 0 0 154863 9.9990631 0 0 0 0 0 0 0 0 0.763323 2564
1.00133 1.00133 2.858 1412 400000 210209 4 2682 2734 2625 0 1 0 0 0 150505 9.9990631 0 0 0 0 0 0 0 0 0.755646 2625
1.00133 1.00133 2.859 1415 400000 212158 1 2689 2734 2637 0 1 0 0 0 152799 9.9990631 0 0 0 0 0 0 0 0 0.762536 2637
1.00133 1.00133 2.86 1418 400000 213132 2 2964 3027 2887 0 1 0 0 0 154230 9.9990631 0 0 0 0 0 0 0 0 0.767447 2887
1.00133 1.00133 2.861 1421 400000 215394 2 2836 2887 2782 0 1 0 0 0 155455 9.9990631 0 0 0 0 0 0 0 0 0.773786 2782
1.00133 1.00133 2.862 1425 400000 217312 0 3122 3166 3060 0 1 0 0 0 157487 9.9990631 0 0 0 0 0 0 0 0 0.780963 3060
1.00133 1.00133 2.863 1428 400000 217329 3 3262 3328 3192 0 1 0 0 0 157812 9.9990631 0 0 0 0 0 0 0 0 0.781131 3192
1.00133 1.00133 2.864 1431 400000 219117 1 3423 3497 3356 0 1 0 0 0 161200 9.9990631 0 0 0 0 0 0 0 0 0.788173 3356
1.00133 1.00133 2.865 1434 400000 218155 4 3490 3559 3411 0 1 0 0 0 158784 9.9990631 0 0 0 0 0 0 0 0 0.784488 3411
1.00133 1.00133 2.866 1437 400000 216532 1 3612 3675 3544 0 1 0 0 0 160833 9.9990631 0 0 0 0 0 0 0 0 0.778812 3544
1.00133 1.00133 2.867 1441 400000 213366 0 3681 3765 3598 0 1 0 0 0 150249 9.9990631 0 0 0 0 0 0 0 0 0.76729 3598
1.00133 1.00133 2.868 1444 400000 213725 1 3715 3786 3642 0 1 0 0 0 153936 9.9990631 0 0 0 0 0 0 0 0 0.768794 3642
1.00133 1.00133 2.869 1447 400000 216163 4 3881 3941 3798 0 1 0 0 0 154307 9.9990631 0 0 0 0 0 0 0 0 0.777622 3798
1.00133 1.00133 2.87 1450 400000 218890 1 4303 4387 4205 0 1 0 0 0 156193 9.9990631 0 0 0 0 0 0 0 0 0.787592 4205
1.00133 1.00133 2.871 1453 400000 218829 1 4271 4346 4187 0 1 0 0 0 154483 9.9990631 0 0 0 0 0 0 0 0 0.787632 4187
1.00133 1.00133 2.872 1457 400000 220899 5 4467 4547 4359 0 1 0 0 0 158051 9.9990631 0 0 0 0 0 0 0 0 0.795457 4359
1.00133 1.00133 2.873 1460 400000 223974 2 4842 4939 4729 0 1 0 0 0 162056 9.9990631 0 0 0 0 0 0 0 0 0.807066 4729
1.00133 1.00133 2.874 1463 400000 223853 3 5001 5097 4895 0 1 0 0 0 160299 9.9990631 0 0 0 0 0 0 0 0 0.806878 4895
1.00133 1.00133 2.875 1466 400000 219871 4 4924 5011 4824 0 1 0 0 0 157386 9.9990631 0 0 0 0 0 0 0 0 0.792261 4824
1.00133 1.00133 2.876 1469 400000 218463 3 4983 5073 4876 0 1 0 0 0 153268 9.9990631 0 0 0 0 0 0 0 0 0.786813 4876
1.00133 1.00133 2.877 1473 400000 217604 3 5094 5177 5002 0 1 0 0 0 157895 9.9990631 0 0 0 0 0 0 0 0 0.78404 5002
1.00133 1.00133 2.878 1476 400000 220096 5 5570 5683 5464 0 1 0 0 0 159735 9.9990631 0 0 0 0 0 0 0 0 0.793091 5464
1.00133 1.00133 2.879 1479 400000 220094 5 5629 5744 5499 0 1 0 0 0 161287 9.9990631 0 0 0 0 0 0 0 0 0.793177 5499
1.00133 1.00133 2.88 1482 400000 223850 6 5961 6074 5832 0 1 0 0 0 162744 9.9990631 0 0 0 0 0 0 0 0 0.807608 5832
1.00133 1.00133 2.881 1486 400000 220236 4 5910 6001 5768 0 1 0 0 0 162567 9.9990631 0 0 0 0 0 0 0 0 0.793738 5768
1.00133 1.00133 2.882 1489 400000 220269 4 6219 6321 6077 0 1 0 0 0 161879 9.9990631 0 0 0 0 0 0 0 0 0.793964 6077
1.00133 1.00133 2.883 1492 400000 217361 4 6208 6316 6091 0 1 0 0 0 158162 9.9990631 0 0 0 0 0 0 0 0 0.783397 6091
1.00133 1.00133 2.884 1495 400000 215963 3 6489 6594 6352 0 1 0 0 0 157681 9.9990631 0 0 0 0 0 0 0 0 0.778338 6352
1.00133 1.00133 2.885 1498 400000 215559 7 6569 6711 6437 0 1 0 0 0 161554 9.9990631 0 0 0 0 0 0 0 0 0.776965 6437
1.00133 1.00133 2.886 1501 400000 217476 5 6766 6872 6621 0 1 0 0 0 158440 9.9990631 0 0 0 0 0 0 0 0 0.783961 6621
1.00133 1.00133 2.887 1505 400000 219494 4 6939 7079 6814 0 1 0 0 0 159418 9.9990631 0 0 0 0 0 0 0 0 0.791666 6814
1.00133 1.00133 2.888 1508 400000 220449 4 7403 7537 7262 0 1 0 0 0 160905 9.9990631 0 0 0 0 0 0 0 0 0.795713 7262
1.00133 1.00133 2.889 1511 400000 216764 5 7434 7555 7267 0 1 0 0 0 158275 9.9990631 0 0 0 0 0 0 0 0 0.782138 7267
1.00133 1.00133 2.89 1514 400000 216278 3 7466 7615 7314 0 1 0 0 0 158145 9.9990631 0 0 0 0 0 0 0 0 0.780219 7314
1.00133 1.00133 2.891 1518 400000 215009 13 7598 7753 7431 0 1 0 0 0 153781 9.9990631 0 0 0 0 0 0 0 0 0.775617 7431
1.00133 1.00133 2.892 1521 400000 216584 10 7693 7836 7530 0 1 0 0 0 154757 9.9990631 0 0 0 0 0 0 0 0 0.781495 7530
1.00133 1.00133 2.893 1524 400000 216772 6 8300 8426 8109 0 1 0 0 0 155548 9.9990631 0 0 0 0 0 0 0 0 0.782269 8109
1.00133 1.00133 2.894 1527 400000 215287 10 8451 8572 8262 0 1 0 0 0 150606 9.9990631 0 0 0 0 0 0 0 0 0.777158 8262
1.00133 1.00133 2.895 1530 400000 212983 7 8267 8394 8100 0 1 0 0 0 155053 9.9990631 0 0 0 0 0 0 0 0 0.76928 8100
1.00133 1.00133 2.896 1534 400000 212760 9 8492 8667 8294 0 1 0 0 0 155905 9.9990631 0 0 0 0 0 0 0 0 0.768586 8294
1.00133 1.00133 2.897 1537 400000 210507 10 8683 8848 8493 0 1 0 0 0 154364 9.9990631 0 0 0 0 0 0 0 0 0.760678 8493
1.00133 1.00133 2.898 1540 400000 208856 13 8841 9014 8641 0 1 0 0 0 151760 9.9990631 0 0 0 0 0 0 0 0 0.754732 8641
1.00133 1.00133 2.899 1543 400000 208609 15 8990 9144 8790 0 1 0 0 0 149256 9.9990631 0 0 0 0 0 0 0 0 0.749576 8790
1.00133 1.00133 2.9 1546 400000 207046 10 9301 9463 9084 0 1 0 0 0 146938 9.9990631 0 0 0 0 0 0 0 0 0.744602 9084
1.00133 1.00133 2.901 1549 400000 207183 17 9478 9641 9267 0 1 0 0 0 150086 9.9990436 0 0 0 0 0 0 0 0 0.745229 9267
1.00133 1.00133 2.902 1553 400000 209064 7 9680 9849 9477 0 1 0 0 0 151895 9.9990631 0 0 0 0 0 0 0 0 0.751224 9477
1.00133 1.00133 2.903 1556 400000 206119 10 9901 10052 9680 0 1 0 0 0 149265 9.9990631 0 0 0 0 0 0 0 0 0.741661 9680
1.00133 1.00133 2.904 1559 400000 206220 13 10184 10363 9953 0 1 0 0 0 149130 9.9990436 0 0 0 0 0 0 0 0 0.741984 9953
1.00133 1.00133 2.905 1562 400000 208649 17 10401 10567 10143 0 1 0 0 0 149428 9.9990436 0 0 0 0 0 0 0 0 0.750146 10143
1.00133 1.00133 2.906 1565 400000 212450 16 10986 11185 10746 0 1 0 0 0 153086 9.9990631 0 0 0 0 0 0 0 0 0.763383 10746
1.00133 1.00133 2.907 1569 400000 211765 22 11289 11466 11015 0 1 0 0 0 152995 9.9990631 0 0 0 0 0 0 0 0 0.761721 11015
1.00133 1.00133 2.908 1572 400000 213365 23 11441 11608 11151 0 1 0 0 0 152739 9.9990631 0 0 0 0 0 0 0 0 0.76681 11151
1.00133 1.00133 2.909 1575 400000 211170 23 11616 11801 11343 0 1 0 0 0 154123 9.9990631 0 0 0 0 0 0 0 0 0.759248 11343
1.00133 1.00133 2.91 1578 400000 211016 12 11855 12063 11579 0 1 0 0 0 152547 9.9990631 0 0 0 0 0 0 0 0 0.7587 11579
1.00133 1.00133 2.911 1581 400000 209611 20 11911 12113 11627 0 1 0 0 0 149865 9.9990631 0 0 0 0 0 0 0 0 0.754012 11627
1.00133 1.00133 2.912 1585 400000 210007 19 12390 12596 12083 0 1 0 0 0 150941 9.9990631 0 0 0 0 0 0 0 0 0.756436 12083
1.00133 1.00133 2.913 1588 400000 211884 24 12632 12848 12315 0 1 0 0 0 154443 9.9990631 0 0 0 0 0 0 0 0 0.762308 12315
1.00133 1.00133 2.914 1591 400000 213157 16 12858 13076 12603 0 1 0 0 0 155177 9.9990631 0 0 0 0 0 0 0 0 0.76676 12603
1.00133 1.00133 2.915 1594 400000 213056 31 13078 13287 12755 0 1 0 0 0 156111 9.9990631 0 0 0 0 0 0 0 0 0.76633 12755
1.00133 1.00133 2.916 1597 400000 215861 23 13948 14153 13629 0 1 0 0 0 156116 9.9990631 0 0 0 0 0 0 0 0 0.776286 13629
1.00133 1.00133 2.917 1601 400000 213724 33 13943 14197 13596 0 1 0 0 0 152412 9.9990631 0 0 0 0 0 0 0 0 0.769135 13596
1.00133 1.00133 2.918 1604 400000 213182 24 14119 14332 13798 0 1 0 0 0 150199 9.9990631 0 0 0 0 0 0 0 0 0.767139 13798
1.00133 1.00133 2.919 1607 400000 215570 29 14738 14975 14360 0 1 0 0 0 155035 9.9990631 0 0 0 0 0 0 0 0 0.775939 14360
1.00133 1.00133 2.92 1610 400000 217483 27 15081 15311 14700 0 1 0 0 0 157602 9.9990631 0 0 0 0 0 0 0 0 0.78316 14700
1.00133 1.00133 2.921 1613 400000 217325 25 15389 15646 15070 0 1 0 0 0 156302 9.9990631 0 0 0 0 0 0 0 0 0.782463 15070
1.00133 1.00133 2.922 1617 400000 215089 30 15188 15408 14798 0 1 0 0 0 153816 9.9990631 0 0 0 0 0 0 0 0 0.774464 14798
1.00133 1.00133 2.923 1620 400000 214769 29 15774 16032 15425 0 1 0 0 0 151230 9.9990631 0 0 0 0 0 0 0 0 0.774272 15425
1.00133 1.00133 2.924 1623 400000 213650 42 15870 16101 15462 0 1 0 0 0 148617 9.9990631 0 0 0 0 0 0 0 0 0.769825 15462
1.00133 1.00133 2.925 1626 400000 216081 52 16558 16801 16153 0 1 0 0 0 151208 9.9990631 0 0 0 0 0 0 0 0 0.778844 16153
1.00133 1.00133 2.926 1629 400000 215730 33 16461 16733 16077 0 1 0 0 0 153621 9.9990631 0 0 0 0 0 0 0 0 0.777123 16077
1.00133 1.00133 2.927 1633 400000 218245 49 17327 17622 16901 0 1 0 0 0 156028 9.9990631 0 0 0 0 0 0 0 0 0.786618 16901
1.00133 1.00133 2.928 1636 400000 220118 53 18076 18349 17607 0 1 0 0 0 158185 9.9990631 0 0 0 0 0 0 0 0 0.793727 17607
1.00133 1.00133 2.929 1639 400000 220084 48 18265 18538 17785 0 1 0 0 0 160188 9.9990631 0 0 0 0 0 0 0 0 0.793627 17785
1.00133 1.00133 2.93 1642 400000 217624 52 18346 18631 17873 0 1 0 0 0 157400 9.9990631 0 0 0 0 0 0 0 0 0.784698 17873
1.00133 1.00133 2.931 1645 400000 214837 45 18488 18749 18032 0 1 0 0 0 157717 9.9990436 0 0 0 0 0 0 0 0 0.7747 18032
1.00133 1.00133 2.932 1649 400000 215193 54 18614 18871 18129 0 1 0 0 0 156889 9.9990436 0 0 0 0 0 0 0 0 0.775885 18129
1.00133 1.00133 2.933 1652 400000 213608 49 18635 18914 18190 0 1 0 0 0 150302 9.9990631 0 0 0 0 0 0 0 0 0.770149 18190
1.00133 1.00133 2.934 1655 400000 215055 50 19326 19602 18820 0 1 0 0 0 151733 9.9990631 0 0 0 0 0 0 0 0 0.775882 18820
1.00133 1.00133 2.935 1658 400000 215759 68 19673 19995 19174 0 1 0 0 0 152803 9.9990436 0 0 0 0 0 0 0 0 0.778406 19174
1.00133 1.00133 2.936 1662 400000 213819 56 19763 20057 19246 0 1 0 0 0 150830 9.9990631 0 0 0 0 0 0 0 0 0.770496 19246
1.00133 1.00133 2.937 1665 400000 213390 46 20271 20550 19731 0 1 0 0 0 146384 9.9990631 0 0 0 0 0 0 0 0 0.766337 19731
1.00133 1.00133 2.938 1668 400000 211587 48 20319 20611 19770 0 1 0 0 0 146389 9.9990631 0 0 0 0 0 0 0 0 0.759232 19770
1.00133 1.00133 2.939 1671 400000 212885 55 20485 20735 19957 0 1 0 0 0 149270 9.9990631 0 0 0 0 0 0 0 0 0.764017 19957
1.00133 1.00133 2.94 1674 400000 212916 57 21251 21562 20663 0 1 0 0 0 149071 9.9990631 0 0 0 0 0 0 0 0 0.764424 20663
1.00133 1.00133 2.941 1678 400000 210837 78 21510 21805 20930 0 1 0 0 0 151622 9.9990631 0 0 0 0 0 0 0 0 0.757636 20930
1.00133 1.00133 2.942 1681 400000 214362 59 22406 22682 21845 0 1 0 0 0 151414 9.9990631 0 0 0 0 0 0 0 0 0.770007 21845
1.00133 1.00133 2.943 1684 400000 213817 79 22698 22976 22067 0 1 0 0 0 151040 9.9990631 0 0 0 0 0 0 0 0 0.768148 22067
1.00133 1.00133 2.944 1687 400000 210987 70 22781 23055 22183 0 1 0 0 0 151607 9.9990631 0 0 0 0 0 0 0 0 0.758462 22183
1.00133 1.00133 2.945 1690 400000 213053 91 23090 23373 22450 0 1 0 0 0 153447 9.9990631 0 0 0 0 0 0 0 0 0.765139 22450
1.00133 1.00133 2.946 1694 400000 213665 77 24230 24570 23499 0 1 0 0 0 154583 9.9990631 0 0 0 0 0 0 0 0 0.767756 23499
1.00133 1.00133 2.947 1697 400000 216307 100 25232 25577 24528 0 1 0 0 0 151875 9.9990631 0 0 0 0 0 0 0 0 0.777474 24528
1.00133 1.00133 2.948 1700 400000 216110 93 26050 26383 25361 0 1 0 0 0 152211 9.9990631 0 0 0 0 0 0 0 0 0.776829 25361
1.00133 1.00133 2.949 1703 400000 219055 86 27007 27314 26245 0 1 0 0 0 159551 9.9990631 0 0 0 0 0 0 0 0 0.788317 26245
1.00133 1.00133 2.95 1706 400000 215357 86 26502 26788 25765 0 1 0 0 0 156283 9.9990631 0 0 0 0 0 0 0 0 0.773619 25765
1.00133 1.00133 2.951 1710 400000 215481 117 27350 27679 26630 0 1 0 0 0 155238 9.9990631 0 0 0 0 0 0 0 0 0.774448 26630
1.00133 1.00133 2.952 1713 400000 214113 129 27341 27648 26515 0 1 0 0 0 156620 9.9990631 0 0 0 0 0 0 0 0 0.769567 26515
1.00133 1.00133 2.953 1716 400000 216518 133 28683 28944 27815 0 1 0 0 0 160495 9.9990631 0 0 0 0 0 0 0 0 0.778673 27815
1.00133 1.00133 2.954 1719 400000 216595 99 29317 29608 28490 0 1 0 0 0 156540 9.9990631 0 0 0 0 0 0 0 0 0.77879 28490
1.00133 1.00133 2.955 1722 400000 218921 127 30529 30878 29665 0 1 0 0 0 155579 9.9990631 0 0 0 0 0 0 0 0 0.787203 29665
1.00133 1.00133 2.956 1726 400000 215519 150 30683 31072 29766 0 1 0 0 0 155383 9.9990436 0 0 0 0 0 0 0 0 0.775293 29766
1.00133 1.00133 2.957 1729 400000 215747 139 31496 31846 30615 0 1 0 0 0 153778 9.9990631 0 0 0 0 0 0 0 0 0.776396 30615
1.00133 1.00133 2.958 1732 400000 213750 135 31794 32129 30831 0 1 0 0 0 151323 9.9990631 0 0 0 0 0 0 0 0 0.76879 30831
1.00133 1.00133 2.959 1735 400000 212146 185 31937 32257 30975 0 1 0 0 0 147423 9.9990631 0 0 0 0 0 0 0 0 0.76297 30975
1.00133 1.00133 2.96 1738 400000 210970 156 32698 33083 31720 0 1 0 0 0 153923 9.9990631 0 0 0 0 0 0 0 0 0.758818 31720
1.00133 1.00133 2.961 1742 400000 208587 144 33096 33414 32078 0 1 0 0 0 150333 9.9990436 0 0 0 0 0 0 0 0 0.750901 32078
1.00133 1.00133 2.962 1745 400000 208911 176 33920 34180 32814 0 1 0 0 0 150866 9.9990631 0 0 0 0 0 0 0 0 0.752252 32814
1.00133 1.00133 2.963 1748 400000 211119 164 35575 35964 34435 0 1 0 0 0 152393 9.9990631 0 0 0 0 0 0 0 0 0.759916 34435
1.00133 1.00133 2.964 1751 400000 213216 188 36779 37085 35596 0 1 0 0 0 154675 9.9990631 0 0 0 0 0 0 0 0 0.767351 35596
1.00133 1.00133 2.965 1754 400000 213402 197 37970 38319 36812 0 1 0 0 0 152879 9.9990631 0 0 0 0 0 0 0 0 0.767994 36812
1.00133 1.00133 2.966 1758 400000 215771 193 39882 40208 38650 0 1 0 0 0 157268 9.9990631 0 0 0 0 0 0 0 0 0.776675 38650
1.00133 1.00133 2.967 1761 400000 217550 241 41629 41977 40288 0 1 0 0 0 157653 9.9990631 0 0 0 0 0 0 0 0 0.783304 40288
1.00133 1.00133 2.968 1764 400000 217611 248 42759 43124 41364 0 1 0 0 0 160806 9.9990631 0 0 0 0 0 0 0 0 0.783547 41364
1.00133 1.00133 2.969 1767 400000 215152 277 43407 43691 41965 0 1 0 0 0 158655 9.9990631 0 0 0 0 0 0 0 0 0.774783 41965
1.00133 1.00133 2.97 1770 400000 215958 299 45489 45769 43885 0 1 0 0 0 155037 9.9990631 0 0 0 0 0 0 0 0 0.777939 43885
1.00133 1.00133 2.971 1774 400000 217670 333 48374 48695 46679 0 1 0 0 0 155338 9.9990631 0 0 0 0 0 0 0 0 0.784347 46679
1.00133 1.00133 2.972 1777 400000 215211 355 48478 48758 46688 0 1 0 0 0 150590 9.9990631 0 0 0 0 0 0 0 0 0.775751 46688
1.00133 1.00133 2.973 1780 400000 215181 379 50751 51059 48838 0 1 0 0 0 152894 9.9990631 0 0 0 0 0 0 0 0 0.775556 48838
1.00133 1.00133 2.974 1783 400000 212711 400 51677 51974 49805 0 1 0 0 0 150698 9.9990631 0 0 0 0 0 0 0 0 0.762322 49805
1.00133 1.00133 2.975 1786 400000 211557 462 54487 54610 52339 0 1 0 0 0 150365 9.9990631 0 0 0 0 0 0 0 0 0.759017 52339
1.00133 1.00133 2.976 1790 400000 211378 488 57374 57598 55148 0 1 0 0 0 150422 9.9990436 0 0 0 0 0 0 0 0 0.75856 55148
1.00133 1.00133 2.977 1793 400000 214318 533 61246 61425 58885 0 1 0 0 0 151825 9.9990631 0 0 0 0 0 0 0 0 0.768513 58885
1.00133 1.00133 2.978 1797 400000 216369 70 22154 22468 66376.766 100 0.32458647 0 0 0 155213 9.9990631 0 0 0 0 0 0 0 0 0.77578 21545
1.00133 1.00133 2.979 1800 400000 217489 83 23774 24091 71361.57 100 0.32458647 0 0 0 158603 9.9990631 0 0 0 0 0 0 0 0 0.77994 23163
1.00133 1.00133 2.98 1804 400000 219115 88 25777 26078 77224.415 100 0.32458647 0 0 0 157628 9.9990436 0 0 0 0 0 0 0 0 0.786028 25066
1.00133 1.00133 2.981 1807 400000 222183 119 28343 28706 84753.995 100 0.32458647 0 0 0 166118 9.9990631 0 0 0 0 0 0 0 0 0.798067 27510
1.00133 1.00133 2.982 1810 400000 221754 125 30690 31030 91722.863 100 0.32458647 0 0 0 161898 9.9990631 0 0 0 0 0 0 0 0 0.796118 29772
1.00133 1.00133 2.983 1813 400000 219409 136 32499 32838 97219.087 100 0.32458647 0 0 0 163035 9.9990631 0 0 0 0 0 0 0 0 0.787591 31556
1.00133 1.00133 2.984 1817 400000 221519 170 36013 36395 107629.26 100 0.32458647 0 0 0 166324 9.9990631 0 0 0 0 0 0 0 0 0.795573 34935
1.00133 1.00133 2.985 1820 400000 221891 208 40216 40527 119940.31 100 0.32458647 0 0 0 163124 9.9990631 0 0 0 0 0 0 0 0 0.797351 38931
1.00133 1.00133 2.986 1823 400000 219515 270 44249 44551 131647.51 100 0.32458647 0 0 0 158346 9.9990631 0 0 0 0 0 0 0 0 0.788354 42731
1.00133 1.00133 2.987 1826 400000 224576 390 52461 52784 155634.95 100 0.32458647 0 0 0 161605 9.9990631 0 0 0 0 0 0 0 0 0.807836 50517
1.00133 1.00133 2.988 1829 400000 223951 492 61113 61325 180900.95 100 0.32458647 0 0 0 161164 9.9990631 0 0 0 0 0 0 0 0 0.805589 58718
1.00133 1.00133 2.989 1834 400000 219756 78 23273 23543 211300.32 200 0.10726912 0 0 0 159390 9.9990631 0 0 0 0 0 0 0 0 0.789307 22666
1.00133 1.00133 2.99 1837 400000 217329 132 31100 31453 281367.08 200 0.10726912 0 0 0 157109 9.9990631 0 0 0 0 0 0 0 0 0.780257 30182
1.00133 1.00133 2.991 1840 400000 217157 231 41394 41737 373182.88 200 0.10726912 0 0 0 159044 9.9990631 0 0 0 0 0 0 0 0 0.779956 40031
1.00133 1.00133 2.992 1844 400000 219481 426 57399 57698 513344.37 200 0.10726912 0 0 0 158676 9.9990631 0 0 0 0 0 0 0 0 0.788631 55066
1.00133 1.00133 2.993 1848 400000 216519 82 26551 26841 742286.22 300 0.034818105 0 0 0 155277 9.9990631 0 0 0 0 0 0 0 0 0.777877 25845
1.00133 1.00133 2.994 1851 400000 214736 219 40560 40942 1125994.6 300 0.034818105 0 0 0 155484 9.9990631 0 0 0 0 0 0 0 0 0.771727 39205
1.00133 1.00133 2.995 1856 400000 212993 87 26558 26850 2055366.7 400 0.012563695 0 0 0 152934 9.9990631 0 0 0 0 0 0 0 0 0.765927 25823
1.00133 1.00133 2.996 1860 400000 212673 135 32026 32365 7635100.7 500 0.0040778768 0 0 0 148809 9.9990631 0 0 0 0 0 0 0 0 0.765014 31135
1.00133 1.00133 2.997 1867 400000 212702 280 46010 46319 2.9112342e+08 800 0.00015247829 0 0 0 154952 9.9990631 0 0 0 0 0 0 0 0 0.765802 44390
1.00133 1.00133 2.998 1870 400000 212709 6 9616 9783 61746497 800 0.00015247829 0 0 0 152313 9.9990631 0 0 0 0 0 0 0 0 0.765923 9415
1.00133 1.00133 2.999 1874 400000 215083 566 61699 61812 3.8816019e+08 800 0.00015247829 0 0 0 157259 9.9990631 0 0 0 0 0 0 0 0 0.774605 59186
1.00133 1.00133 3 1877 400000 212531 131 28126 28471 1.793698e+08 800 0.00015247829 0 0 0 158718 9.9990631 0 0 0 0 0 0 0 0 0.765256 27350
1.00133 1.00133 3.001 1881 400000 210612 90 25258 25560 56075941 700 0.00043742467 0 0 0 150228 9.9990631 0 0 0 0 0 0 0 0 0.758256 24529
1.00133 1.00133 3.002 1887 400000 212932 11 11921 12101 2856788.9 500 0.0040780053 0 0 0 158781 9.9990631 0 0 0 0 0 0 0 0 0.766295 11650
1.00133 1.00133 3.003 1892 400000 210836 28 14863 15094 1156984.5 400 0.012563695 0 0 0 155849 9.9990631 0 0 0 0 0 0 0 0 0.758871 14536
1.00133 1.00133 3.004 1896 400000 208124 67 20011 20292 560024.73 300 0.034818105 0 0 0 153364 9.9990631 0 0 0 0 0 0 0 0 0.749535 19499
1.00133 1.00133 3.005 1899 400000 208376 14 11159 11342 313802.26 300 0.034818105 0 0 0 154939 9.9990631 0 0 0 0 0 0 0 0 0.750745 10926
1.00133 1.00133 3.006 1904 400000 211018 76 22310 22585 202350.87 200 0.10726912 0 0 0 149825 9.9990631 0 0 0 0 0 0 0 0 0.755339 21706
1.00133 1.00133 3.007 1907 400000 212226 42 15902 16131 144505.7 200 0.10726912 0 0 0 152900 9.9990436 0 0 0 0 0 0 0 0 0.759828 15501
1.00133 1.00133 3.008 1910 400000 212296 24 12210 12361 111131.7 200 0.10726912 0 0 0 151527 9.9990631 0 0 0 0 0 0 0 0 0.760047 11921
1.00133 1.00133 3.009 1913 400000 209527 8 9632 9798 87984.313 200 0.10726912 0 0 0 148857 9.9990631 0 0 0 0 0 0 0 0 0.750712 9438
1.00133 1.00133 3.01 1916 400000 208222 11 7826 7982 71409.18 200 0.10726912 0 0 0 154517 9.9990631 0 0 0 0 0 0 0 0 0.746763 7660
1.00133 1.00133 3.011 1921 400000 208400 47 19339 19599 58095.459 100 0.32458647 0 0 0 150084 9.9990631 0 0 0 0 0 0 0 0 0.747176 18857
1.00133 1.00133 3.012 1924 400000 209593 31 15753 15981 47466.551 100 0.32458647 0 0 0 151502 9.9990436 0 0 0 0 0 0 0 0 0.751307 15407
1.00133 1.00133 3.013 1927 400000 212445 29 13687 13903 41073.801 100 0.32458647 0 0 0 155487 9.9990631 0 0 0 0 0 0 0 0 0.761379 13332
1.00133 1.00133 3.014 1930 400000 212388 21 11838 12036 35611.466 100 0.32458647 0 0 0 153023 9.9990631 0 0 0 0 0 0 0 0 0.761351 11559
1.00133 1.00133 3.015 1933 400000 213902 11 10405 10548 31390.711 100 0.32458647 0 0 0 155386 9.9990631 0 0 0 0 0 0 0 0 0.767174 10189
1.00133 1.00133 3.016 1937 400000 211560 12 8774 8902 26430.553 100 0.32458647 0 0 0 152932 9.9990631 0 0 0 0 0 0 0 0 0.75907 8579
1.00133 1.00133 3.017 1940 400000 210798 9 7581 7688 22841.371 100 0.32458647 0 0 0 153925 9.9990631 0 0 0 0 0 0 0 0 0.755881 7414
1.00133 1.00133 3.018 1944 400000 208867 45 19645 19861 19130 0 1 0 0 0 151642 9.9990436 0 0 0 0 0 0 0 0 0.74935 19130
1.00133 1.00133 3.019 1947 400000 211771 50 18029 18309 17572 0 1 0 0 0 155935 9.9990631 0 0 0 0 0 0 0 0 0.759381 17572
1.00133 1.00133 3.02 1951 400000 211499 48 16130 16343 15733 0 1 0 0 0 154153 9.9990631 0 0 0 0 0 0 0 0 0.758782 15733
1.00133 1.00133 3.021 1954 400000 213865 32 14689 14891 14316 0 1 0 0 0 151453 9.9990631 0 0 0 0 0 0 0 0 0.767057 14316
1.00133 1.00133 3.022 1957 400000 215906 22 13372 13594 13003 0 1 0 0 0 152339 9.9990436 0 0 0 0 0 0 0 0 0.774726 13003
1.00133 1.00133 3.023 1960 400000 216466 19 12229 12439 11913 0 1 0 0 0 152965 9.9990436 0 0 0 0 0 0 0 0 0.777313 11913
1.00133 1.00133 3.024 1963 400000 219405 15 11558 11766 11301 0 1 0 0 0 157917 9.9990436 0 0 0 0 0 0 0 0 0.788551 11301
1.00133 1.00133 3.025 1966 400000 215564 14 10139 10365 9915 0 1 0 0 0 154074 9.9990436 0 0 0 0 0 0 0 0 0.773809 9915
1.00133 1.00133 3.026 1970 400000 215655 11 9282 9433 9065 0 1 0 0 0 155111 9.9990631 0 0 0 0 0 0 0 0 0.77446 9065
1.00133 1.00133 3.027 1973 400000 213613 7 8603 8773 8423 0 1 0 0 0 154231 9.9990436 0 0 0 0 0 0 0 0 0.767254 8423
1.00133 1.00133 3.028 1976 400000 214817 9 7891 8044 7697 0 1 0 0 0 154939 9.9990631 0 0 0 0 0 0 0 0 0.771787 7697
1.00133 1.00133 3.029 1979 400000 216578 15 7523 7664 7332 0 1 0 0 0 154421 9.9990631 0 0 0 0 0 0 0 0 0.77903 7332
1.00133 1.00133 3.03 1982 400000 213631 8 6924 7046 6754 0 1 0 0 0 152217 9.9990631 0 0 0 0 0 0 0 0 0.76721 6754
1.00133 1.00133 3.031 1986 400000 213598 5 6484 6600 6357 0 1 0 0 0 155069 9.9990631 0 0 0 0 0 0 0 0 0.767029 6357
1.00133 1.00133 3.032 1989 400000 211047 4 6019 6132 5903 0 1 0 0 0 149947 9.9990631 0 0 0 0 0 0 0 0 0.75835 5903
1.00133 1.00133 3.033 1992 400000 213140 2 5622 5732 5518 0 1 0 0 0 151626 9.9990631 0 0 0 0 0 0 0 0 0.766317 5518
1.00133 1.00133 3.034 1995 400000 213250 2 5299 5392 5197 0 1 0 0 0 151245 9.9990436 0 0 0 0 0 0 0 0 0.767067 5197
#C Wed Jun 16 18:57:54 2010. Scan aborted after 185 points.