Parameter Editor#
A parameter dictionary can be edited with a
ParameterEditor()
widget.
See also
Here is one example.
View of a parameter dictionary using ParameterEditor
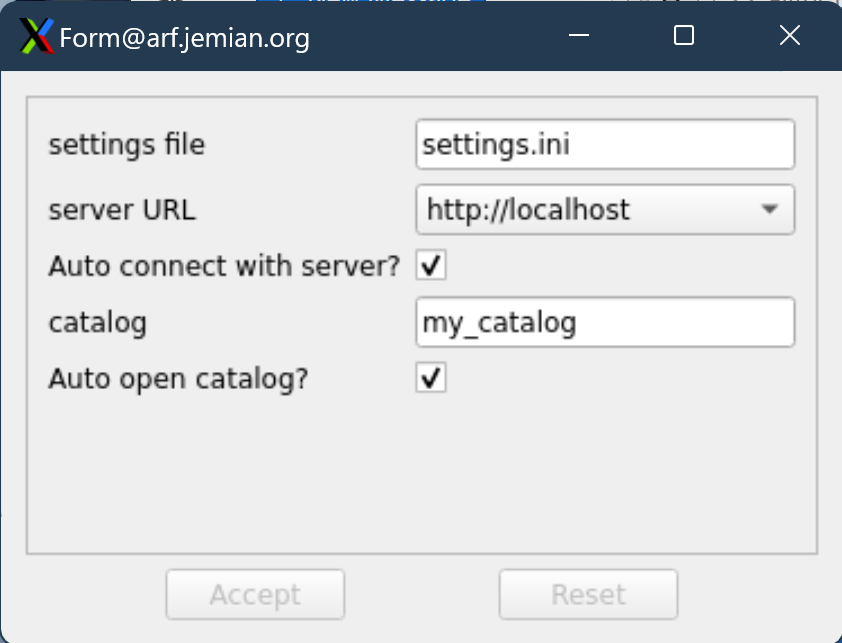
View of ParameterEditor
dialog.#
Full Python application to display parameters in a ParameterEditor widget
"""Parameters Editor Demo Application"""
import sys
from PyQt5 import QtWidgets
import pyQParamWidget as qpw
# build the dictionary
parameters = {
"settings_file": qpw.ParameterItemText(
label="settings file", value="settings.ini"
),
"server": qpw.ParameterItemChoice(
label="server URL",
value="http://localhost",
choices=[
"http://localhost",
"http://localhost.localdoman",
"http://127.0.0.1",
],
),
"autoconnect": qpw.ParameterItemCheckbox(
label="Autoconnect with server?", value=True
),
"catalog": qpw.ParameterItemText(label="catalog", value="my_catalog"),
"autoopen": qpw.ParameterItemCheckbox(
label="Auto open catalog?", value=True
),
}
# Show ParameterEditor in a PyQt application
app = QtWidgets.QApplication(sys.argv)
window = qpw.ParameterEditor(None, parameters)
window.show()
print(f"{window.values()=}")
sys.exit(app.exec())
For the source code documentation, see
ParameterEditor
.
EXAMPLE
First, make a dictionary of
ParameterItem
objects.
The keys of the dictionary can be strings or Python objects or
any other structure allowed by Python as dictionary keys. The
keys, themselves, are not used by ParameterEditor
. They
are only used to identify each of the ParameterItem
objects.
This example defines four objects:
1parameters = {
2 "item1": qpw.param_item.ParameterItemText(
3 "title",
4 "Suggested title",
5 tooltip="Set the title. Be brief."
6 ),
7 "item2": qpw.param_item.ParameterItemChoice(
8 "gain scale",
9 "10 uA/V",
10 choices=["1 mA/V", "10 uA/V", "100 nA/V", "100 nA/V"],
11 tooltip="Pick a gain scale.",
12 ),
13 "item3": qpw.param_item.ParameterItemCheckbox(
14 "wait",
15 True,
16 tooltip="Should wait at each point?",
17 ),
18 "item4": qpw.param_item.ParameterItemSpinBox(
19 "# of points",
20 21,
21 lo=2, hi=10_000,
22 tooltip="How many points to collect?",
23 ),
24}
Next, create the ParameterEditor
object, passing in the parent
object (usually the QWidget
object that will contain this new widget) and
the parameters
dictionary.
editor = ParameterEditor(parent, parameters)
Finally, add editor
into parent’s layout.
Get the values from the editor widget#
The widget’s values()
method returns a dictionary with the accepted parameter values. The dictionary
keys are the same as the parameter dictionary supplied.
editor = ParameterEditor(parent, parameters)
# ...user interaction
results = editor.values()
EXAMPLE
Using the parameters
dictionary above, and making no changes in the editor window,
print(results)
would return:
{
"item1": "Suggested title",
"item2": "10 uA/V",
"item3": True,
"item4": 21
}
Data types#
The editor attempts to report the values in the original data type as given in the dictionary of parameters.