Parameter Tree#
A hierarchy of parameters can be edited using the
ParameterTree()
dialog.
See also
Here is one example.
View of a hierarchical parameter dictionary using ParameterTree
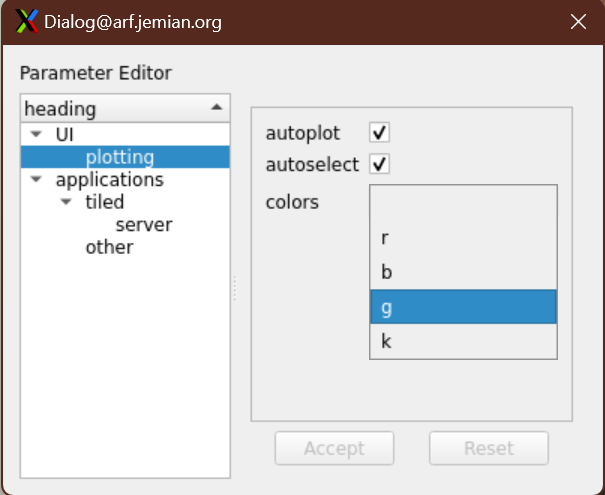
View of ParameterTree
dialog.#
The hierarchy (a dictionary of dictionaries) is displayed as a tree on the left side. The keys are text strings, to be displayed in the tree. The end of each branch of the tree is a dictionary of Parameter Items. See the next block of Python code. When the end of a branch is selected, a Parameter Editor is shown in the right side of the dialog.
Python code to construct the example hierarchical parameter dictionary
1import pyQParamWidget as qpw
2
3hierarchy = {
4 "applications": {
5 "tiled": {
6 "server": {
7 "settings_file": qpw.ParameterItemText(
8 label="settings file", value="~/.config/settings.ini"
9 ),
10 "catalog": qpw.ParameterItemText(label="catalog", value="bluesky_data"),
11 "url": qpw.ParameterItemText(label="url", value="http://localhost"),
12 },
13 },
14 "other": {
15 "demo": qpw.ParameterItemCheckbox("demo mode?", True),
16 },
17 },
18 "UI": {
19 "plotting": {
20 "autoplot": qpw.ParameterItemCheckbox(
21 label="autoplot",
22 value=True,
23 tooltip="Plot when the run is selected.",
24 ),
25 "autoselect": qpw.ParameterItemCheckbox(
26 label="autoselect",
27 value=True,
28 tooltip="Automatically select the signals to plot.",
29 ),
30 "colors": qpw.ParameterItemChoice(
31 label="colors", value="", choices=["", "r", "b", "g", "k"]
32 ),
33 },
34 },
35}
Python code to display the hierarchy in a ParameterTree dialog
1dialog = qpw.ParameterTree(None, parameters=hierarchy)
2# dialog.show() # modeless: does not block
3dialog.exec() # modal: blocks
4# Show the final values of the parameters, once the dialog is closed.
5print(f"{dialog.values()=}")
Get the values from the tree dialog#
The widget’s values()
method returns a hierarchical dictionary with the accepted parameter values. The dictionary
keys are the same as the parameter dictionary (hierarchy
) supplied.
dialog = qpw.ParameterTree(None, parameters=hierarchy)
dialog.exec()
# ...user interaction
results = dialog.values()
EXAMPLE
Using the hierarchy
dictionary above, and making no changes in the tree dialog,
print(results)
would return:
{
"applications": {
"tiled": {
"server": {
"settings_file": "~/.config/settings.ini",
"catalog": "bluesky_data",
"url": "http://localhost",
},
},
"other": {
"demo": True,
},
},
"UI": {
"plotting": {
"autoplot": True,
"autoselect": True,
"colors": "",
},
}