Parameter Items#
An individual parameter item has several pieces of information, as described
in the source code documentation, see
ParameterItemBase
. There are different
types, depending on the type of parameter to be edited. Here is an example of a
dictionary of Parameter Items.
Example dictionary of Parameter Items
1import pyQParamWidget as qpw
2parms = {
3 "title": qpw.ParameterItemText("title", "Suggested title"),
4 "color": qpw.ParameterItemChoice(
5 "color",
6 "",
7 choices=["", "red", "green", "blue"],
8 tooltip="Pick a color.",
9 ),
10 "autoscale": qpw.ParameterItemCheckbox(
11 "autoscale",
12 True,
13 tooltip="Otherwise, not autoscale.",
14 ),
15}
The Parameter Item types are listed next:
ParameterItemCheckbox#
ParameterItemCheckbox
uses a QCheckBox
widget to edit a boolean parameter.
ParameterItemCheckbox(
"autoscale", True, tooltip="Otherwise, not autoscale."
)
Example widget to edit a ParameterItemCheckbox.

Example widget to edit a ParameterItemCheckbox
.#
ParameterItemChoice#
ParameterItemChoice
uses a QComboBox
widget to select a value from a list of choices.
ParameterItemChoice(
"color", "",
choices=["", "red", "green", "blue"],
tooltip="Pick a color.",
)
Example widget to edit a ParameterItemChoice.
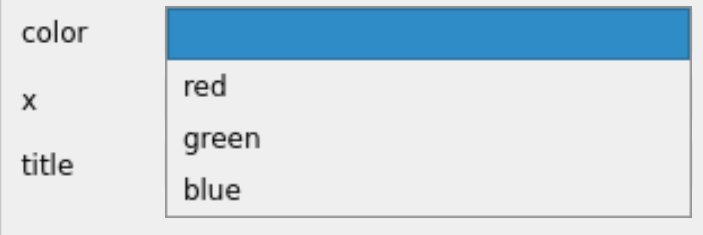
Example widget to edit a ParameterItemChoice
. Drop-down
menu is selected to show the list of choices.#
ParameterItemSpinBox#
ParameterItemSpinBox
uses a QSpinBox
widget to select a value within limits of lo
and hi
.
ParameterItemSpinBox(
"x", 50,
hi=100,
lo=0,
tooltip="Choose a value from the range.",
)
Example widget to edit a ParameterItemSpinBox.

Example widget to edit a ParameterItemSpinBox
.#
ParameterItemText#
ParameterItemText
uses a QLineEdit
widget to edit a value as text.
ParameterItemText("title", "Suggested title", tooltip="Set the title. Be brief.")
Example widget to edit a ParameterItemText.

Example widget to edit a ParameterItemText
. The tooltip is also shown.#